FB.setAccessToken is not a function
setAccessToken. This is a non-standard api and does not exist in the official client side FB JS SDK. Warning: Due to Node's asynchronous nature, you should not use setAccessToken when FB is used on behalf of for multiple users.
Regex match text between tags
Source: https://stackoverflow.com/questions/11592033/regex-match-text-between-tags
/<b>(.*?)<\/b>/g
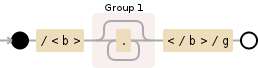
Add
g
(global) flag after:/<b>(.*?)<\/b>/g.exec(str)
//^-----here it is
However if you want to get all matched elements, then you need something like this:
var str = "<b>Bob</b>, I'm <b>20</b> years old, I like <b>programming</b>.";
var result = str.match(/<b>(.*?)<\/b>/g).map(function(val){
return val.replace(/<\/?b>/g,'');
});
//result -> ["Bob", "20", "programming"]
Chain multiple Node http request
Source: https://stackoverflow.com/questions/34835940/chain-multiple-node-http-request
Firstly, you'll want to take a look at request, which is most popular choice for HTTP requests, due to its simplicity.
Secondly, we can combine the simplicity of request with the concept of Promises, to make multiple requests in succession, while keeping the code flat.
Using request-promise
Using request-promise
var rp = require('request-promise')
var url1 = {}
var url2 = {}
var url3 = {}
rp(url1)
.then(response => {
// add stuff from url1 response to url2
return rp(url2)
})
.then(response => {
// add stuff from url2 response to url3
return rp(url3)
})
.then(response => {
// do stuff after all requests
// If something went wrong
// throw new Error('messed up')
})
.catch(err => console.log) // Don't forget to catch errors
As you can see, we can add as many requests as we want, and the code will stay flat and simple. As a bonus, we were able to add error-handling as well. Using traditional callbacks, you'd have to add error-handling to every callback, whereas here we only have to do it once at the end of the Promise chain.
UPDATE (09/16): While Promises take us halfway there, further experience has convinced me that Promises alone get messy when there is a lot of mixing between sync, async code, and especially control flow (e.g. if-else). The canonical way to solve this would be with async/await, however that is still in development and would require transpilation. As such, generators are the next best solution.
Using co
var co = require('co')
var rp = require('request-promise')
var url1 = {}
var url2 = {}
var url3 = {}
co(function* () {
var response
response = yield rp(url1)
// add stuff from url1 response to url2
response = yield rp(url2)
// add stuff from url2 response to url3
response = yield rp(url3)
// do stuff after all requests
// If something went wrong
// throw new Error('messed up')
})
.catch(err => console.log) // Don't forget to catch errors
UPDATE (12/16): Now that the latest version of node at time of writing (7.2.1) supports async/await behind the
--harmony
flag, you could do this:const rp = require('request-promise')
const url1 = {}
const url2 = {}
const url3 = {}
async function doRequests() {
let response
response = await rp(url1)
// add stuff from url1 response to url2
response = await rp(url2)
// add stuff from url2 response to url3
response = await rp(url3)
// do stuff after all requests
// If something went wrong
// throw new Error('messed up')
}
doRequests()
.catch(err => console.log) // Don't forget to catch errors
Đăng ký:
Bài đăng (Atom)
Cold Turkey Blocker
https://superuser.com/questions/1366153/how-to-get-rid-of-cold-turkey-website-blocker-get-around-the-block Very old question, but still wan...
-
Basic usage | Documentation | Poetry - Python dependency management and packaging made easy (python-poetry.org) Activating the virtual env...
-
Basic usage | Documentation | Poetry - Python dependency management and packaging made easy (python-poetry.org) Python: poetry create poet...
-
Optional chaining (?.) - JavaScript | MDN (mozilla.org) The optional chaining operator ( ?. ) enables you to read the value of a propert...